Why use HTML buttons in Phaser 3? Normally I use images for buttons in Phaser, but if I’m using other form elements, like input text, sometimes it makes sense to use HTML buttons. It also allows me to use CSS styles on the buttons. This can be important because many times I’m handed designs by UI designers who don’t understand the difference between canvas and non-canvas design.
Adding the buttons
Building on the first part of this series, I’ve added two HTML form buttons in the HTML with the ids of ‘btnSend’ and ‘btnCancel’. I’ve also added some bootstrap classes for stlying
<body>
<div id="phaser-game">
</div>
<input id="myText" type="text"/>
<textarea id="area51">
SOME TEXT HERE
</textarea>
<button class="btn btn-success" id="btnSend" name="btnSend">
Send
</button>
<button class="btn btn-danger" id="btnCancel" name="btnCancel">
Cancel
</button>
</body>
Adding Bootstrap
Next for the styling I’ve linked in the bootstrap from a CDN between the head tags
<link crossorigin="anonymous" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity="sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" rel="stylesheet">
Scaling and Placing
Now for the Phaser part, we can use the same functions we used for lining up and scaling the input text, for the buttons. In sceneMain’s create function add:
this.formUtil.scaleToGameW("btnSend", .25);
this.formUtil.placeElementAt(101, "btnSend");
this.formUtil.scaleToGameW("btnCancel", .25);
this.formUtil.placeElementAt(107, "btnCancel");
Result
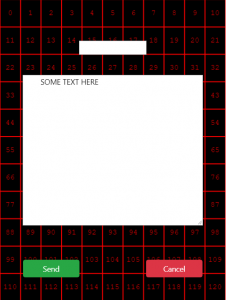
Adding Callbacks to the buttons
While we could just use simple JavaScript to call functions, I decided to create a function in the FormUtil.js to speed things up, and bring it more in line with the style we are already using in Phaser. In the formUtil.js add the following function:
addClickCallback(elName, fun, scope = null) {
var el = document.getElementById(elName);
if (scope == null) {
el.onclick = fun;
} else {
el.onclick = fun.bind(scope);
}
}
The addClickCallback function takes an element id that is passed as a string. It gets the element from the document based on the id and then adds a click event linking to the function to that element. If a scope is passed then it binds the scope to the function. Here is how we can use it in sceneMain (bottom of create function)
this.formUtil.addClickCallback("btnSend", this.sendForm, this);
this.formUtil.addClickCallback("btnCancel", this.cancelForm, this);
And then the functions we will be calling
sendForm() {
console.log("sendForm");
}
cancelForm() {
console.log("cancelForm");
}
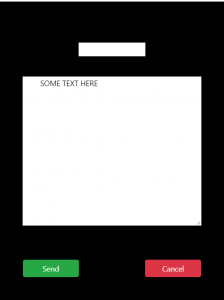
This is an awesome example, but how were you able to make the html elements only show in the Phaser game screen, and not in the webpage itself too?
Well, they are on the webpage as well. They just overlay the canvas.