Using ladders in Phaser 3 is tricky. Not only do you need to detect when a player touches the ladder, but you also need to know when it stops touching as well.
The technique that I’ve decided to use here, will allow us to call a function to check if any ladder is being touched by the character at any given time.
This is part of the series on making platform games in Phaser 3. This tutorial also uses the Utility Template which helps scale and align objects in Phaser 3.
Placing the ladder
Preload the image
First, let’s preload the ladder image into the library.
this.load.image("ladder", "images/objects/ladder.png");
Add the ladder group
Next, add a group to hold all the ladder in the game. This will make detecting a ladder easier since we only have to check the ninja against the group.
this.ladderGroup = this.physics.add.group();
Place the ladder on the grid
Next, we create a function to place all objects on the grid. We just need to send a position, a key for the image, and a type. If the type is “ladder” then we add that to the ladderGroup.
makeObj(pos, key, type) {
let obj = this.physics.add.image(0, 0, key);
Align.scaleToGameW(obj, .1);
this.blockGrid.placeAtIndex(pos, obj);
if (type == "ladder") {
this.ladderGroup.add(obj);
}
}
Then call the function that will place the ladder on the grid.
this.makeObj(378, "ladder", "ladder");
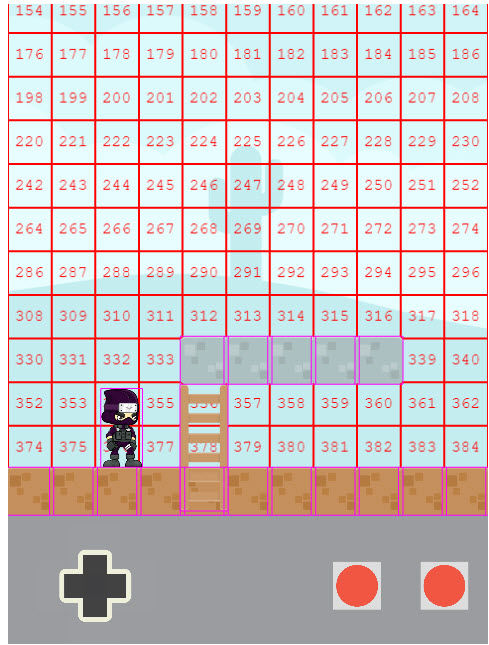
Detecting the ladder
My first thought was to use something like the following code.
this.physics.add.collider(this.ninja, this.ladderGroup,this.onLadder);
The problem was the ninja kept kicking the ladder out of the way. If I made the ladder immovable then the ninja couldn’t get on the ladder at all.
Using Overlap
The solution was a two-step process. First I changed collider to overlap.
this.physics.add.overlap(this.ninja, this.ladderGroup);
What is the difference between collisions and overlap in phaser 3?
Overlap doesn’t cause the objects to interact with each other but causes triggers in Phaser when two objects overlap. I could add a function to the overlap, but it would only trigger when I touched the ladder. There is not any way I know to set up a function in overlap or collider to call a function when something has stopped colliding or overlapping.
Using Touching
Here is where the second part comes in. Touching.
sprite.body.touching.none
When used with the overlap check on physics, the touching.none will equal true when nothing is touching the bounding box. If something is touching then it will be false.
It is a double negative and easy to get confused, but just remember if it is “not none” then you have an overlap.
So when we need to check if a sprite is on the ladder, we just need to loop through the ladders and see if it is touching anything. Since we are only setting the overlap between the ladders and the player then if something is touching the ladder, we know the ninja is on the ladder.
checkLadder()
{
this.onLadder=false;
this.ladderGroup.children.iterate(function(child)
{
if (!child.body.touching.none)
{
this.onLadder=true;
}
}.bind(this));
}
After calling this function if the variable this.onLadder is equal to true, then you can allow the player to climb the ladder.
Here is what the code will look like on our controlled pressed function from the last tutorial.
controllPressed(param) {
switch (param) {
case "GO_UP":
this.checkLadder();
if (this.onLadder==true)
{
this.ninja.setVelocityY(-250);
}
}