TileMaps in Phaser 3 are very popular with developers because they allow us to rapidly build worlds for our players to explore. Not just in Phaser! Most modern game frameworks support TileMaps.
I’ll admit I’ve had trouble with tileMaps in Phaser 3 before. I kept giving up and writing code to get around them. I finally sat down with some coffee and dove in. I’m not sure what my stumbling block was, but I finally got past it. They are quite easy when you come down to it.
I found the best way was breaking down the parts.
I’ve also included some tileMap snippets to help get you started
Parts of a TileMap
The map
The map object is the top parent object and holds all the other parts of the tileMap. To create a tileMap we need to give dimensions and data to build the the map.
const map = this.make.tilemap({ data:array, tileWidth: 64, tileHeight: 64});
TileSets
Tileset means the collection of tiles that you want to use in the map. This is usually in the form of a png spritesheet image with a transparent background. We add it to the map object with the following code:
map.addTilesetImage("tiles");
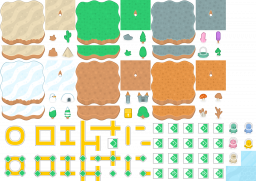
Layers
A layer is an object where you will actually place the tiles. You can have multiple layers. As the name suggests you can place one layer on top of another. This allows you arrange the z-order of the tiles, so you can place some objects above or below other. It also allows us to detect collisions only from certain layers.
const layer = map.createLayer(0, "tiles", 0, 0);
Data
The data consist of either an array or a key to preloaded file (JSON or CVS). In the example below we have an array of sub-arrays. Each number denotes a tile image or keyframe of the tileSet. Each array is a row, and each entry a column.
const array=[[0,1,2,22],
[17,18,19],
[34,35,36]];
Making a map with a multi-dimensional array
Here is how looks all together:
export class SceneMain extends Phaser.Scene {
constructor() {
super("SceneMain");
}
preload() {
this.load.image("tiles","src/assets/tiles.png");
}
create() {
//make a multi-dimensonial array
const array=[[0,1,2,22],
[17,18,19],
[34,35,36]];
//make the tile map
const map = this.make.tilemap({ data:array, tileWidth: 64, tileHeight: 64});
//add the tileset to the map
map.addTilesetImage("tiles");
//add a layer to the map
const layer = map.createLayer(0, "tiles", 0, 0);
}
update() {
}
}
export default SceneMain;
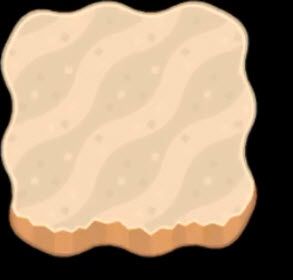